Creating a Customer (Individual) via API
This endpoint enables you to create individual customers. Once a customer is created, we initiate the KYC verification process automatically. You can then either poll the customer object or listen for webhooks to track the status change from PENDING_AGREEMENTS
→ VERIFIED
or REJECTED
.
How Embedded Partner Customer creation works
Embedded Wallets allow Embedded Partners (EP) to offer seamless payment and fund management capabilities directly within their platform while maintaining their branding. This guide provides a step-by-step process to create Embedded Partner Customer (EPC) accounts utilising Embedded Wallets via the BVNK Portal.
This product is available to businesses and individuals based in the US, EEA and other countries supported by BVNK outside of these regions.
EPC State transition diagram
The creation and verification lifecycle of an EPC follows a series of states—Pending Agreements, Info Required, Pending, Verified, and Rejected—which are illustrated and explained in this section.
Understanding these states (and how an EPC transitions between them) is key to a smooth onboarding process.
- Pending Agreements
- The EPC has been created but must consent to BVNK’s Terms of Service.
- An agreements link is generated and must be shared with the EPC to collect consent.
- Info Required
- After Terms are signed, BVNK requires additional verification information (KYB/KYC).
- A verification link is provided for the EP to provide their Customer's (EPC) data and documents.
- Pending
- Once verification flow is submitted, BVNK performs compliance checks.
- During this time, you can monitor the EPC’s status in the portal.
- Verified
- The EPC has successfully passed the compliance review.
- Wallets can now be created and used.
- Rejected
- The EPC has failed compliance checks or provided invalid information.
- The flow halts unless additional data is requested and submitted for reconsideration.
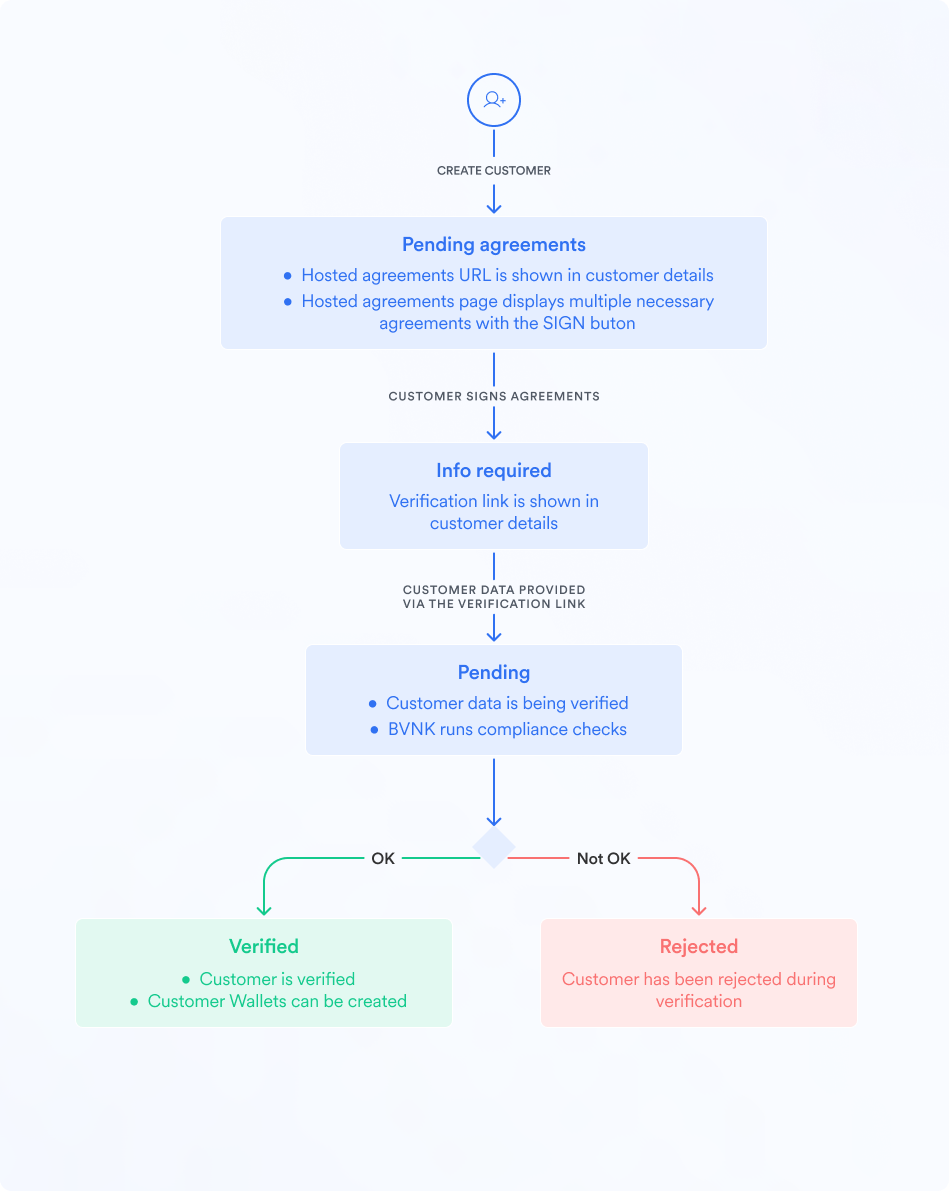
Important
- Ensure accurate personal data (name, DOB, nationality, address) is collected to facilitate a smooth KYC process.
- A successful response includes a unique customer reference for tracking verification status.
- Customer Due Diligence (CDD) data is required.
Early Access
Please note that this endpoint is currently in early access, and it may undergo changes as we continue to improve and refine the functionality.
Prerequisites
You will need to collect information about the customer before proceeding with the request to create one.
Idempotency supported endpoint
This means you can retry a request without worrying about it being processed multiple times.
To use this feature, add the
X-Idempotency-Key
header with a unique value (a UUID). This ensures the request is only processed once, even if it's sent multiple times.
Create an Individual Customer
Endpoints
Environment | Endpoint |
---|---|
Production | POST https://api.bvnk.com/platform/v1/customers |
Sandbox | POST https://api.sandbox.bvnk.com/platform/v1/customers |
Request
Example - individual customer creation:
{
"type": "INDIVIDUAL",
"riskScore": "LOW",
"individual": {
"description": "Transfers to/from Own Wallet (including Bank Accounts)",
"firstName": "John",
"lastName": "Doe",
"dateOfBirth": "1990-05-12",
"nationality": "US",
"birthCountryCode": "US",
"emailAddress": "[email protected]",
"phoneNumber": "+1234567890",
"address": {
"addressLine1": "123 Main St",
"city": "New York",
"postalCode": "10001",
"stateCode": "NY",
"countryCode": "US"
},
"taxIdentification": {
"number": "123-45-6789",
"taxResidenceCountryCode": "US"
},
"cdd": {
"employmentStatus": "Salaried",
"sourceOfFunds": "Salary / Employment Income",
"pepStatus": "Not a PEP",
"expectedMonthlyVolume": {
"amount": "500.01",
"currency": "EUR"
}
}
},
"signedAgreementSessionReference": "78eedde1-2402-4a59-8bbd-ccecb6d612d1"
}
Request Body Parameters (Individual)
Attribute | Type | Required | Description |
---|---|---|---|
type | string | Yes | Must be INDIVIDUAL . |
individual | object | Yes | Individual customer details |
individual.riskScore | string | Yes | Customer risk rating (LOW , MEDIUM , HIGH ) |
individual.description | string | Yes | Purpose (e.g., Transfers, Investments, Donations) |
individual.firstName | string | Yes | First name |
individual.lastName | string | Yes | Last name |
individual.dateOfBirth | string | Yes | YYYY-MM-DD format |
individual.nationality | string | Optional | Nationality (ISO 2-letter code) |
individual.contactinfo | object | Yes | Contact information |
individual.contactinfo.email | string | Yes | Email address |
individual.contactinfo.phonenumber | string | Optional | Phone number (optional) |
individual.address | object | Yes | Customer Residence Address |
individual.address.addressLine1 | string | Yes | Street Name and Number |
individual.address.addressLine2 | string | Optional | Additional street information |
individual.address.city | string | Yes | City |
individual.address.postCode | string | Yes | ZIP/postal code |
individual.address.stateCode | string | Optional | State. Required for US customers |
individual.address.countryCode | string | Yes | Country ISO 2-letter code |
individual.taxidentification | object | Yes | Tax Identification details |
individual.taxidentification.number | string | Yes | SSN/ITIN for US, Tax number for Rest of the world |
individual.taxidentification.taxResidenceCountryCode | string | Yes | Tax residency country ISO 2-letter code |
individual.cdd | object | Yes | Customer Due Diligence details |
individual.cdd.employmentStatus | string | Yes | Employment status. One of the following: Self-employed/Freelancer , Salaried , Unemployed , Retired , Not Provided |
individual.cdd.sourceOfFunds | string | Yes | Source of funds. One of the following: Salary/Employment Income , Pension/Retirement Income , Savings&Investments , Self-Employment/Business Income , Crypto Trading , Gambling/Lottery Winnings , Real Estate Sales |
individual.cdd.pepStatus | string | Yes | Politically Exposed Person status (one of the following: Not a PEP , Former/Inactive PEP (within 2 years) , Former/Inactive PEP (older than 2 years) , Domestic PEP , Foreign PEP , Close Associates , Family Members ) |
individual.cdd.expectedMonthlyVolume | object | Yes | Expected monthly volume object |
individual.cdd.expectedMonthlyVolume.amount | string | Yes | Expected Monthly Volume amount |
individual.cdd.expectedMonthlyVolume.currency | string | Optional | USD or EUR ,USD by default |
signedAgreementSessionReference | string | Yes | Agreement acceptance reference |
Response
Response Code | Description |
---|---|
202 | Successful operation. |
400 | Bad request. |
{
"reference": "f81a5b62-27b8-4b3b-b51e-eb10edeb1731",
"status": "INFO_REQUIRED"
}
{
"code": "ACCOUNTS-2000",
"status": "BAD_REQUEST",
"message": "Invalid request",
"details": {
"errors": {
"signedAgreementSessionReference": [
"Agreement session with reference: 814d949b-9a6d-49ea-92f5-23ee51b37e24 is already assigned to the customer"
]
}
}
}
Attribute | Type | Description |
---|---|---|
reference | string | A unique identifier for the customer. |
status | string | The current status of the customer. Possible values include: - PENDING_AGREEMENTS - pending customer agreement consent,- INFO_REQUIRED - customer information is needed for verification,- PENDING - pending customer verification,- VERIFIED - customer has been verified,- REJECTED - verification has been unsuccessful. |
Add Documents to an Individual Customer
- Use these endpoints to attach documents to an Individual customer.
- Each request can upload multiple documents simultaneously.
Important
- Reference the correct
customerReference
.- Files must be base64-encoded before sending.
Endpoints
Add Document(s)
Environment | Endpoint |
---|---|
Production | POST https://api.bvnk.com/platform/v1/customers/{customerReference}/documents |
Sandbox | POST https://api.sandbox.bvnk.com/platform/v1/customers/{customerReference}/documents |
Request Example
{
"customerPersonReference": "9b2c3d4e-567f-48f0-abc1-03a4e3df12ab",
"type": "PASSPORT",
"subType": "FRONT_SIDE",
"name": "John Smith Passport.pdf",
"description": "Document optional description",
"countryCode": "GB",
"externalReference": "2b8d8bde-eef5-408a-9228-96bef24865ad",
"content": "JVBERi0xLjUKJeLjz9MKMSAwIG9iago8PC9UeXBlIC9QYWdl..."
}
Path Parameter
Parameter | Type | Required | Description |
---|---|---|---|
customerReference | string | Yes | The unique identifier of the customer to whom the documents will be attached. Customer has to be in INFO_REQUIRED status (status after agreement consent) |
Request Body Parameters
Attribute | Type | Required | Description |
---|---|---|---|
customerPersonReference | string(36) | No | Links the document to a specific associate (e.g., beneficial owner). If omitted or null , the document is attached at the company level. |
type | string | Yes | The primary document type, e.g. PASSPORT , DRIVERS_LICENSE , COMPANY_DOC Full list of supported document types |
subType | string | No | Additional specification of the document type, e.g. FRONT_SIDE , BACK_SIDE if applicable.Full list of supported document subTypes |
name | string(128) | Yes | Optional human-readable filename, e.g. "John Smith Passport.pdf" . |
description | string(255) | No | Optional description for the provided document |
countryCode | string(2) | Yes | ISO 3166-1 alpha-2 country code associated with the document. |
externalReference | string(36) | Yes | The internal reference mapped to the document from your end. |
content | string | Yes | Base64-encoded file content. |
Response
Response Code | Description |
---|---|
202 | Request accepted and documents queued for upload. |
400 | Bad request (e.g., missing or invalid data). |
Success Example:
{
"reference": "d42e1c62-27b8-4b3b-b51e-eb10edeb1731",
"externalReference": "2b8d8bde-eef5-408a-9228-96bef24865ad",
"status": "INIT"
}
Failure Example:
{
"code": "DOCUMENTS-4000",
"status": "BAD_REQUEST",
"message": "Invalid document content",
"details": {
"errors": {
"content": [
"Document file content is not valid base64"
]
}
}
}
Additional Document APIs
Search Documents
GET /platform/v1/customers/documents
Optional Query Parameters:
customerReference
externalReference
name
types
subTypes
statuses
(e.g., INIT, PENDING, APPROVED, DECLINED)
Get Document Download URL
GET /platform/v1/customers/documents/{documentReference}/url
Returns a temporary URL for document download.
Delete Document
DELETE /platform/v1/customers/documents/{documentReference}
Returns 204 No Content
if successfully deleted.
Good to Know
-
Check Document Status
Retrieve the customer or associated person object to confirm document verification status. -
Retain Document References
Store thereference
returned in the success response for future queries or audits. -
Combine with KYC
Document verification may be required before the customer status changes fromPENDING
toVERIFIED
.
Complete Onboarding
Once you have uploaded all required documentation for an Embedded Partner Customer (EPC), you must confirm the completion of the onboarding process by calling the following endpoint:
Endpoint
Environment | Method | URL |
---|---|---|
Production | POST | https://api.bvnk.com/platform/v1/customers/{reference}/complete-onboarding |
Sandbox | POST | https://api.sandbox.bvnk.com/platform/v1/customers/{reference}/complete-onboarding |
Path Parameters
Parameter | Type | Required | Description |
---|---|---|---|
reference | string | Yes | The unique reference identifier for the Embedded Partner Customer (EPC) provided during customer creation. |
What Happens Next?
When you successfully call this endpoint:
- BVNK will initiate final compliance and verification checks.
- In the sandbox environment, EPC verification is automatically approved.
- In production, if the EPC meets all compliance requirements, the status will update to
VERIFIED
. If there are outstanding requirements or issues, the status will move toINFO_REQUIRED
orREJECTED
, prompting manual review or further actions.
Monitoring Status
You can monitor the EPC's verification status via:
- API: Retrieve current status using
GET /platform/v1/customers/{reference}
- Portal: Track progress and statuses directly through the BVNK Portal
- Webhooks: Set up webhooks to receive automated updates on status changes.
Updated 10 minutes ago