Creating a Webhook Listener
BVNK sends webhooks for status changes on payment transactions, so it's a good idea to set up a listener in your service to get the most out of our Crypto Payments API.
You can specify your Webhook URL inside the settings of each Merchant you create on the BVNK platform.
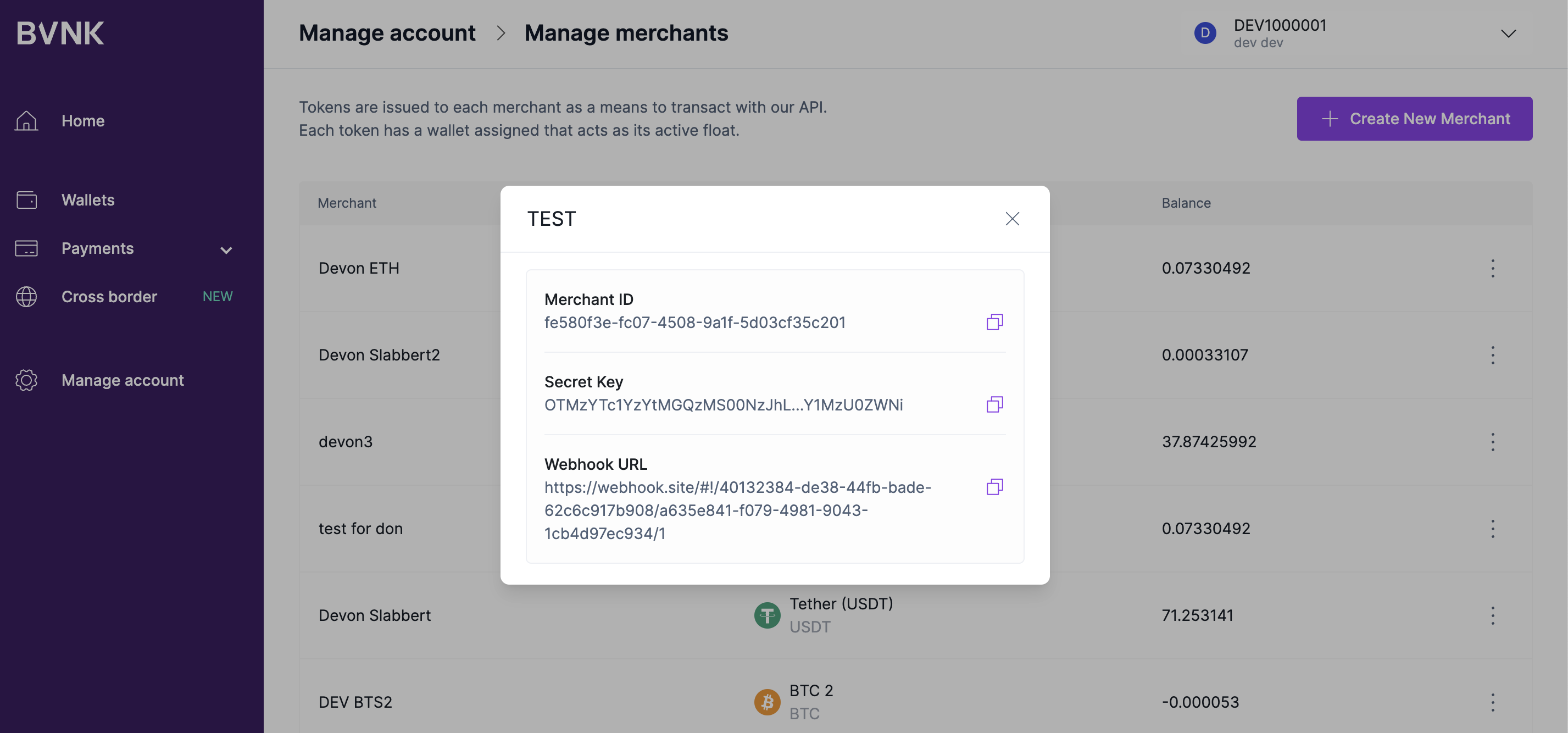
Change the URL here at anytime.
Webhooks will be sent as an HTTP POST with Content-Type: application/json
containing the payload of the object.
We recommend that you always check the status of the payment on the API after you receive a webhook as further details may be required, such as the final amount paid for the transaction you have been notified about.
You should stop updating transactions in your system after receiving the final webhook from BVNK - this prevents duplicate transaction IDs from affecting your customers.
Acknowledging events
The Crypto Payments product is expecting a 200
status code upon receipt of a webhook that has been successfully sent.
If your webhook script performs any logic upon receiving a webhook, you should return a
200
status code before completing the logic to prevent timeouts.
Webhook retry policy
If BVNK do not receive a 200
status code, the webhook retry policy will kick in.
The webhook retry policy works by adding a delay before each retry, starting with a base delay and increasing the time between retries. The delay grows larger after each attempt but won't go beyond 15 minutes. The system will try up to 100 times, and after that, no more retries will happen.
Handling duplicate events
Callback endpoints might occasionally receive the same event more than once. We advise you to guard against duplicated event receipts. One way of doing this is logging the events you’ve processed, and then not processing the logged ones.
Webhook validation
Webhooks you receive from the Crypto Payments product contain a signature to allow servers to verify the authenticity of the request using a server-side secret key.
How the signature is calculated
- Concatenate the webhook url, content-type and payload of the webhook
- Generate the signature using the Secret Key and hashedBody components. The secret key can be obtained in your BVNK merchant profile under Settings -> Manage Merchants -> Merchant Name
Below are examples in some popular programming languages
Be careful!
Make sure you do not parse your HTTP request into an object and then serialize it.
Instead, just get the webhook raw payload as a string.
import org.apache.commons.codec.digest.HmacAlgorithms;
import org.apache.commons.codec.digest.HmacUtils;
import java.net.URI;
import java.net.URISyntaxException;
/**
* This is a utility class used for validating webhooks from the Crypto Payments product.
* It provides a way to verify the authenticity of the incoming webhook request.
*/
public class Main {
/**
* This is the main method which drives the process of webhook validation.
* @param args Unused.
* @throws URISyntaxException If the provided webhook URL is malformed.
*/
public static void main(String[] args) throws URISyntaxException {
//EXAMPLE TEST DATA
/* Secret key that can be found under Settings -> Manage Merchants -> Merchant Name
in your BVNK merchant account
*/
String secretKey = "LWExNWYtYjRjYzJiZjRmZGZhNDU5NmFjNWMtYjhmNS00OTFhLWE0YTAtMWUwZjU5YjAwZjJk";
/* Webhook URL that you've set under Settings -> Manage Merchants -> Webhook URL */
String webhookUrl = "https://webhook.site/23dcv4a41-d4935-4e16-9ed6-ce812f7bddcd8";
/* Content-Type needed for concatenating the values */
String contentType = "application/json";
/* Body of the webhook. Make sure the webhook payload is escaped correctly with no whitespaces*/
String payload = "{\"source\":\"payment\",\"event\":\"statusChanged\",\"data\":{\"uuid\":\"e605bdf8-ef54-456a-909c-07af117239c7\",\"merchantDisplayName\":\"Fortuna ETH\",\"merchantId\":\"f0006b3a-3fda-47c6-ac21-367a482f3499\",\"dateCreated\":1685022891000,\"expiryDate\":1685195689000,\"quoteExpiryDate\":1685023804000,\"acceptanceExpiryDate\":1685022924000,\"quoteStatus\":\"PAYMENT_IN_RECEIVED\",\"reference\":\"REF319526\",\"type\":\"OUT\",\"subType\":\"merchantPayOut\",\"status\":\"PROCESSING\",\"displayCurrency\":{\"currency\":\"ETH\",\"amount\":0.02,\"actual\":0.02},\"walletCurrency\":{\"currency\":\"ETH\",\"amount\":0.02,\"actual\":0.02},\"paidCurrency\":{\"currency\":\"ETH\",\"amount\":0.02,\"actual\":0.020000000000000000},\"feeCurrency\":{\"currency\":\"ETH\",\"amount\":0.0002,\"actual\":0.0002},\"displayRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1},\"exchangeRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1},\"address\":{\"protocol\":null,\"address\":\"0x2588a1002Cc4d7EEC1333bE459471571bbe2EE36\",\"tag\":null,\"uri\":null,\"alternatives\":[]},\"redirectUrl\":\"https://pay.sandbox.bvnk.com/payout?uuid=e605bdf8-ef54-456a-909c-07af117239c7\",\"returnUrl\":\"\",\"transactions\":[{\"dateCreated\":1685022909993,\"dateConfirmed\":null,\"hash\":null,\"amount\":0.020000000000000000,\"risk\":{\"level\":\"LOW\",\"resourceName\":\"UNKNOWN\",\"resourceCategory\":\"UNKNOWN\",\"alerts\":[]},\"networkFeeCurrency\":\"ETH\",\"networkFeeAmount\":0.000000000000000000,\"sources\":null,\"exchangeRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1},\"displayRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1}}],\"refund\":null,\"refunds\":[]}}";
// Concatenate the webhook URL, Content-Type, and payload
String hashBody = getBodyToHash(webhookUrl, contentType, payload);
// Generate the signature
String signature = generateSignature(secretKey, hashBody);
// Output the signature
System.out.println("Generated signature: " + signature);
}
/**
* This method creates a concatenated string from the provided webhook URL, content type, and payload.
* @param webhookUrl The URL where the webhook will be sent.
* @param contentType The MIME type of the content.
* @param payload The actual content body of the webhook.
* @return A concatenated string of the provided parameters.
* @throws URISyntaxException If the provided webhook URL is malformed.
*/
public static String getBodyToHash(String webhookUrl, String contentType, String payload) throws URISyntaxException {
URI uri = new URI(webhookUrl);
StringBuilder hashBody = new StringBuilder();
hashBody.append(uri.getPath());
if (uri.getRawQuery() != null) {
hashBody.append(uri.getRawQuery());
}
if (contentType != null) {
hashBody.append(contentType);
}
if (payload != null) {
hashBody.append(payload);
}
return hashBody.toString();
}
/**
* This method generates a signature from the provided secret and the hashed body.
* It uses the HMAC-SHA256 hashing algorithm.
* @param secret The secret key used to generate the hash.
* @param hashBody The body that needs to be hashed.
* @return The HMAC-SHA256 hash of the body, encoded as a hexadecimal string.
*/
public static String generateSignature(final String secret, String hashBody) {
return toHex(new HmacUtils(HmacAlgorithms.HMAC_SHA_256, secret).hmac(hashBody));
}
/**
* This is a helper method that converts a byte array to a hexadecimal string.
* @param bytes The byte array that needs to be converted.
* @return The hexadecimal representation of the byte array.
*/
static String toHex(byte[] bytes) {
StringBuilder sb = new StringBuilder();
for (byte b : bytes) {
sb.append(String.format("%02x", b));
}
return sb.toString();
}
}
import hashlib
import hmac
import urllib.parse
def get_body_to_hash(webhook_url, content_type, payload):
"""
This method creates a concatenated string from the provided webhook URL, content type, and payload.
:param webhook_url: The URL where the webhook will be sent.
:param content_type: The MIME type of the content.
:param payload: The actual content body of the webhook.
:return: A concatenated string of the provided parameters.
"""
request_url = urllib.parse.urlparse(webhook_url)
body_to_hash = f"{request_url.path}{content_type}{payload}"
return body_to_hash
def generate_signature(secret_key, hash_body):
"""
This method generates a signature from the provided secret and the hashed body.
It uses the HMAC-SHA256 hashing algorithm.
:param secret_key: The secret key used to generate the hash.
:param hash_body: The body that needs to be hashed.
:return: The HMAC-SHA256 hash of the body, encoded as a hexadecimal string.
"""
hashed = hmac.new(secret_key.encode(), hash_body.encode(), hashlib.sha256)
return hashed.hexdigest()
def validate_webhook():
"""
This function prints out the signature from inputs.
"""
# Secret key that can be found under Settings -> Manage Merchants -> Merchant Name in your BVNK merchant account
secret_key = 'YTczZmM5YTEtZDFhNi00YWU5LTk4ODktMmEzODI1NzBkMDI2ZWFjNDcwNzgtNTRkYi00OTY0LWI5NjAtMzRmOTQ0ZTE5NDQ3'
# Webhook URL that you've set under Settings -> Manage Merchants -> Webhook URL
webhook_url = 'https://webhook.site/11488aba-735e-4906-9809-f1a11a91f49f'
# Content-Type needed for concatenating the values
content_type = 'application/json'
# Body of the webhook.
payload = '{"source":"payment","event":"statusChanged","data":{"uuid":"83e96598-dd76-471c-a990-57a64468c436","merchantDisplayName":"Webhooks testing merchant","merchantId":"7cc7256f-124e-4b0b-aad4-251e131c0977","dateCreated":1685004317000,"expiryDate":1685177117000,"quoteExpiryDate":1685177117000,"acceptanceExpiryDate":1685004365000,"quoteStatus":"ACCEPTED","reference":"REF971604","type":"OUT","subType":"merchantPayOut","status":"COMPLETE","displayCurrency":{"currency":"EUR","amount":22.5,"actual":22.5},"walletCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"paidCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"feeCurrency":{"currency":"ETH","amount":0.00014,"actual":0.00014},"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1},"address":{"address":"0x84A4a239805d06c685219801B82BEA7c76702214","tag":null,"protocol":null,"uri":"ethereum:0x84A4a239805d06c685219801B82BEA7c76702214?value=1.352773E+16","alternatives":[]},"returnUrl":"","redirectUrl":"https://pay.sandbox.bvnk.com/payout?uuid=83e96598-dd76-471c-a990-57a64468c436","transactions":[{"dateCreated":1685004367300,"dateConfirmed":1685004367300,"hash":"0x2ac8db80e1d18a15656a564c3de7f085f1a42005cfa4d6fab9a2439ab7bd6f76","amount":0.01352773,"risk":null,"networkFeeCurrency":"ETH","networkFeeAmount":0.00000000,"sources":[],"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1}}],"refund":null,"refunds":[]}}'
# Concatenate the webhook URL, Content-Type, and payload
hash_body = get_body_to_hash(webhook_url, content_type, payload)
# Generate the signature
signature = generate_signature(secret_key, hash_body)
# Output the signature
print(f"Generated signature: {signature}")
validate_webhook()
<?php
/**
* This method creates a concatenated string from the provided webhook URL, content type, and payload.
* @param string $webhookUrl The URL where the webhook will be sent.
* @param string $contentType The MIME type of the content.
* @param string $payload The actual content body of the webhook.
* @return string A concatenated string of the provided parameters.
*/
function getBodyToHash($webhookUrl, $contentType, $payload) {
$requestURL = parse_url($webhookUrl);
$bodyToHash = $requestURL['path'] . $contentType . $payload;
return $bodyToHash;
}
/**
* This method generates a signature from the provided secret and the hashed body.
* It uses the HMAC-SHA256 hashing algorithm.
* @param string $secret The secret key used to generate the hash.
* @param string $hashBody The body that needs to be hashed.
* @return string The HMAC-SHA256 hash of the body, encoded as a hexadecimal string.
*/
function generateSignature($secretKey, $hashBody) {
$hash = hash_hmac('sha256', $hashBody, $secretKey);
return $hash;
}
/**
* This function prints out the signature from inputs.
*/
function validateWebhook() {
// EXAMPLE TEST DATA
$secretKey = 'YTczZmM5YTEtZDFhNi00YWU5LTk4ODktMmEzODI1NzBkMDI2ZWFjNDcwNzgtNTRkYi00OTY0LWI5NjAtMzRmOTQ0ZTE5NDQ3';
$webhookUrl = 'https://webhook.site/11488aba-735e-4906-9809-f1a11a91f49f';
$contentType = 'application/json';
$payload = '{"source":"payment","event":"statusChanged","data":{"uuid":"83e96598-dd76-471c-a990-57a64468c436","merchantDisplayName":"Webhooks testing merchant","merchantId":"7cc7256f-124e-4b0b-aad4-251e131c0977","dateCreated":1685004317000,"expiryDate":1685177117000,"quoteExpiryDate":1685177117000,"acceptanceExpiryDate":1685004365000,"quoteStatus":"ACCEPTED","reference":"REF971604","type":"OUT","subType":"merchantPayOut","status":"COMPLETE","displayCurrency":{"currency":"EUR","amount":22.5,"actual":22.5},"walletCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"paidCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"feeCurrency":{"currency":"ETH","amount":0.00014,"actual":0.00014},"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1},"address":{"address":"0x84A4a239805d06c685219801B82BEA7c76702214","tag":null,"protocol":null,"uri":"ethereum:0x84A4a239805d06c685219801B82BEA7c76702214?value=1.352773E+16","alternatives":[]},"returnUrl":"","redirectUrl":"https://pay.sandbox.bvnk.com/payout?uuid=83e96598-dd76-471c-a990-57a64468c436","transactions":[{"dateCreated":1685004367300,"dateConfirmed":1685004367300,"hash":"0x2ac8db80e1d18a15656a564c3de7f085f1a42005cfa4d6fab9a2439ab7bd6f76","amount":0.01352773,"risk":null,"networkFeeCurrency":"ETH","networkFeeAmount":0.00000000,"sources":[],"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1}}],"refund":null,"refunds":[]}}';
// Concatenate the webhook URL, Content-Type, and payload
$hashBody = getBodyToHash($webhookUrl, $contentType, $payload);
// Generate the signature
$signature = generateSignature($secretKey, $hashBody);
// Output the signature
echo "Generated signature: " . $signature;
}
validateWebhook();
?>
const CryptoJS = require("crypto-js");
/**
* This method creates a concatenated string from the provided webhook URL, content type, and payload.
* @param webhookUrl The URL where the webhook will be sent.
* @param contentType The MIME type of the content.
* @param payload The actual content body of the webhook.
* @return A concatenated string of the provided parameters.
*/
function getBodyToHash(webhookUrl, contentType, payload) {
const requestURL = new URL(webhookUrl);
const bodyToHash = `${requestURL.pathname}${contentType}${payload}`;
return bodyToHash;
}
/**
* This method generates a signature from the provided secret and the hashed body.
* It uses the HMAC-SHA256 hashing algorithm.
* @param secret The secret key used to generate the hash.
* @param hashBody The body that needs to be hashed.
* @return The HMAC-SHA256 hash of the body, encoded as a hexadecimal string.
*/
function generateSignature(secretKey, hashBody) {
const hasher = CryptoJS.HmacSHA256(hashBody, secretKey);
const hashInHex = CryptoJS.enc.Hex.stringify(hasher);
return hashInHex;
}
/**
* This function prints out the signature from inputs.
*/
function validateWebhook(){
// EXAMPLE TEST DATA
// Secret key that can be found under Settings -> Manage Merchants -> Merchant Name in your BVNK merchant account
const secretKey = 'YTczZmM5YTEtZDFhNi00YWU5LTk4ODktMmEzODI1NzBkMDI2ZWFjNDcwNzgtNTRkYi00OTY0LWI5NjAtMzRmOTQ0ZTE5NDQ3';
// Webhook URL that you've set under Settings -> Manage Merchants -> Webhook URL
const webhookUrl = 'https://webhook.site/11488aba-735e-4906-9809-f1a11a91f49f';
// Content-Type needed for concatenating the values
const contentType = 'application/json';
// Body of the webhook.
const payload = '{"source":"payment","event":"statusChanged","data":{"uuid":"83e96598-dd76-471c-a990-57a64468c436","merchantDisplayName":"Webhooks testing merchant","merchantId":"7cc7256f-124e-4b0b-aad4-251e131c0977","dateCreated":1685004317000,"expiryDate":1685177117000,"quoteExpiryDate":1685177117000,"acceptanceExpiryDate":1685004365000,"quoteStatus":"ACCEPTED","reference":"REF971604","type":"OUT","subType":"merchantPayOut","status":"COMPLETE","displayCurrency":{"currency":"EUR","amount":22.5,"actual":22.5},"walletCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"paidCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"feeCurrency":{"currency":"ETH","amount":0.00014,"actual":0.00014},"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1},"address":{"address":"0x84A4a239805d06c685219801B82BEA7c76702214","tag":null,"protocol":null,"uri":"ethereum:0x84A4a239805d06c685219801B82BEA7c76702214?value=1.352773E+16","alternatives":[]},"returnUrl":"","redirectUrl":"https://pay.sandbox.bvnk.com/payout?uuid=83e96598-dd76-471c-a990-57a64468c436","transactions":[{"dateCreated":1685004367300,"dateConfirmed":1685004367300,"hash":"0x2ac8db80e1d18a15656a564c3de7f085f1a42005cfa4d6fab9a2439ab7bd6f76","amount":0.01352773,"risk":null,"networkFeeCurrency":"ETH","networkFeeAmount":0.00000000,"sources":[],"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1}}],"refund":null,"refunds":[]}}';
// Concatenate the webhook URL, Content-Type, and payload
let hashBody = getBodyToHash(webhookUrl, contentType, payload);
// Generate the signature
let signature = generateSignature(secretKey, hashBody);
// Output the signature
console.log(`Generated signature: ${signature}`);
}
validateWebhook();
using System;
using System.Security.Cryptography;
using System.Text;
using System.Web;
class Program
{
static void Main()
{
ValidateWebhook();
}
/*
* This method creates a concatenated string from the provided webhook URL, content type, and payload.
*/
static string GetBodyToHash(string webhookUrl, string contentType, string payload)
{
Uri requestUrl = new Uri(webhookUrl);
string bodyToHash = $"{requestUrl.AbsolutePath}{contentType}{payload}";
return bodyToHash;
}
/*
* This method generates a signature from the provided secret and the hashed body.
* It uses the HMAC-SHA256 hashing algorithm.
*/
static string GenerateSignature(string secretKey, string hashBody)
{
using (var hmac = new HMACSHA256(Encoding.ASCII.GetBytes(secretKey)))
{
var hash = hmac.ComputeHash(Encoding.ASCII.GetBytes(hashBody));
return BitConverter.ToString(hash).Replace("-", "").ToLower();
}
}
/*
* This function prints out the signature from inputs.
*/
static void ValidateWebhook()
{
// Secret key that can be found under Settings -> Manage Merchants -> Merchant Name in your BVNK merchant account
const string secretKey = "YTczZmM5YTEtZDFhNi00YWU5LTk4ODktMmEzODI1NzBkMDI2ZWFjNDcwNzgtNTRkYi00OTY0LWI5NjAtMzRmOTQ0ZTE5NDQ3";
// Webhook URL that you've set under Settings -> Manage Merchants -> Webhook URL
const string webhookUrl = "https://webhook.site/11488aba-735e-4906-9809-f1a11a91f49f";
// Content-Type needed for concatenating the values
const string contentType = "application/json";
// Body of the webhook.
const string payload = "{\"source\":\"payment\",\"event\":\"statusChanged\",\"data\":{\"uuid\":\"83e96598-dd76-471c-a990-57a64468c436\",\"merchantDisplayName\":\"Webhooks testing merchant\",\"merchantId\":\"7cc7256f-124e-4b0b-aad4-251e131c0977\",\"dateCreated\":1685004317000,\"expiryDate\":1685177117000,\"quoteExpiryDate\":1685177117000,\"acceptanceExpiryDate\":1685004365000,\"quoteStatus\":\"ACCEPTED\",\"reference\":\"REF971604\",\"type\":\"OUT\",\"subType\":\"merchantPayOut\",\"status\":\"COMPLETE\",\"displayCurrency\":{\"currency\":\"EUR\",\"amount\":22.5,\"actual\":22.5},\"walletCurrency\":{\"currency\":\"ETH\",\"amount\":0.01353,\"actual\":0.01353},\"paidCurrency\":{\"currency\":\"ETH\",\"amount\":0.01353,\"actual\":0.01353},\"feeCurrency\":{\"currency\":\"ETH\",\"amount\":0.00014,\"actual\":0.00014},\"displayRate\":{\"base\":\"ETH\",\"counter\":\"EUR\",\"rate\":1663.25022749567},\"exchangeRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1},\"address\":{\"address\":\"0x84A4a239805d06c685219801B82BEA7c76702214\",\"tag\":null,\"protocol\":null,\"uri\":\"ethereum:0x84A4a239805d06c685219801B82BEA7c76702214?value=1.352773E+16\",\"alternatives\":[]},\"returnUrl\":\"\",\"redirectUrl\":\"https://pay.sandbox.bvnk.com/payout?uuid=83e96598-dd76-471c-a990-57a64468c436\",\"transactions\":[{\"dateCreated\":1685004367300,\"dateConfirmed\":1685004367300,\"hash\":\"0x2ac8db80e1d18a15656a564c3de7f085f1a42005cfa4d6fab9a2439ab7bd6f76\",\"amount\":0.01352773,\"risk\":null,\"networkFeeCurrency\":\"ETH\",\"networkFeeAmount\":0.00000000,\"sources\":[],\"displayRate\":{\"base\":\"ETH\",\"counter\":\"EUR\",\"rate\":1663.25022749567},\"exchangeRate\":{\"base\":\"ETH\",\"counter\":\"ETH\",\"rate\":1}}],\"refund\":null,\"refunds\":[]}}";
// Concatenate the webhook URL, Content-Type, and payload
string hashBody = GetBodyToHash(webhookUrl, contentType, payload);
// Generate the signature
string signature = GenerateSignature(secretKey, hashBody);
// Output the signature
Console.WriteLine($"Generated signature: {signature}");
}
}
require 'openssl'
require 'uri'
require 'json'
# This method creates a concatenated string from the provided webhook URL, content type, and payload.
# @param webhook_url [String] The URL where the webhook will be sent.
# @param content_type [String] The MIME type of the content.
# @param payload [String] The actual content body of the webhook.
# @return [String] A concatenated string of the provided parameters.
def get_body_to_hash(webhook_url, content_type, payload)
request_url = URI.parse(webhook_url)
body_to_hash = "#{request_url.path}#{content_type}#{payload}"
return body_to_hash
end
# This method generates a signature from the provided secret and the hashed body.
# It uses the HMAC-SHA256 hashing algorithm.
# @param secret_key [String] The secret key used to generate the hash.
# @param hash_body [String] The body that needs to be hashed.
# @return [String] The HMAC-SHA256 hash of the body, encoded as a hexadecimal string.
def generate_signature(secret_key, hash_body)
digest = OpenSSL::Digest.new('sha256')
hmac = OpenSSL::HMAC.hexdigest(digest, secret_key, hash_body)
return hmac
end
# This function prints out the signature from inputs.
def validate_webhook
# EXAMPLE TEST DATA
# Secret key that can be found under Settings -> Manage Merchants -> Merchant Name in your BVNK merchant account
secret_key = 'YTczZmM5YTEtZDFhNi00YWU5LTk4ODktMmEzODI1NzBkMDI2ZWFjNDcwNzgtNTRkYi00OTY0LWI5NjAtMzRmOTQ0ZTE5NDQ3'
# Webhook URL that you've set under Settings -> Manage Merchants -> Webhook URL
webhook_url = 'https://webhook.site/11488aba-735e-4906-9809-f1a11a91f49f'
# Content-Type needed for concatenating the values
content_type = 'application/json'
# Body of the webhook.
payload = '{"source":"payment","event":"statusChanged","data":{"uuid":"83e96598-dd76-471c-a990-57a64468c436","merchantDisplayName":"Webhooks testing merchant","merchantId":"7cc7256f-124e-4b0b-aad4-251e131c0977","dateCreated":1685004317000,"expiryDate":1685177117000,"quoteExpiryDate":1685177117000,"acceptanceExpiryDate":1685004365000,"quoteStatus":"ACCEPTED","reference":"REF971604","type":"OUT","subType":"merchantPayOut","status":"COMPLETE","displayCurrency":{"currency":"EUR","amount":22.5,"actual":22.5},"walletCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"paidCurrency":{"currency":"ETH","amount":0.01353,"actual":0.01353},"feeCurrency":{"currency":"ETH","amount":0.00014,"actual":0.00014},"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1},"address":{"address":"0x84A4a239805d06c685219801B82BEA7c76702214","tag":null,"protocol":null,"uri":"ethereum:0x84A4a239805d06c685219801B82BEA7c76702214?value=1.352773E+16","alternatives":[]},"returnUrl":"","redirectUrl":"https://pay.sandbox.bvnk.com/payout?uuid=83e96598-dd76-471c-a990-57a64468c436","transactions":[{"dateCreated":1685004367300,"dateConfirmed":1685004367300,"hash":"0x2ac8db80e1d18a15656a564c3de7f085f1a42005cfa4d6fab9a2439ab7bd6f76","amount":0.01352773,"risk":null,"networkFeeCurrency":"ETH","networkFeeAmount":0.00000000,"sources":[],"displayRate":{"base":"ETH","counter":"EUR","rate":1663.25022749567},"exchangeRate":{"base":"ETH","counter":"ETH","rate":1}}],"refund":null,"refunds":[]}}'
# Concatenate the webhook URL, Content-Type, and payload
hash_body = get_body_to_hash(webhook_url, content_type, payload)
# Generate the signature
signature = generate_signature(secret_key, hash_body)
# Output the signature
puts "Generated signature: #{signature}"
end
validate_webhook
The signature will be available in the header of the webhook, x-signature
.
Every new webhook will have a new value of the x-signature header. Make sure you are comparing the hash to the right header.
Updated 4 months ago